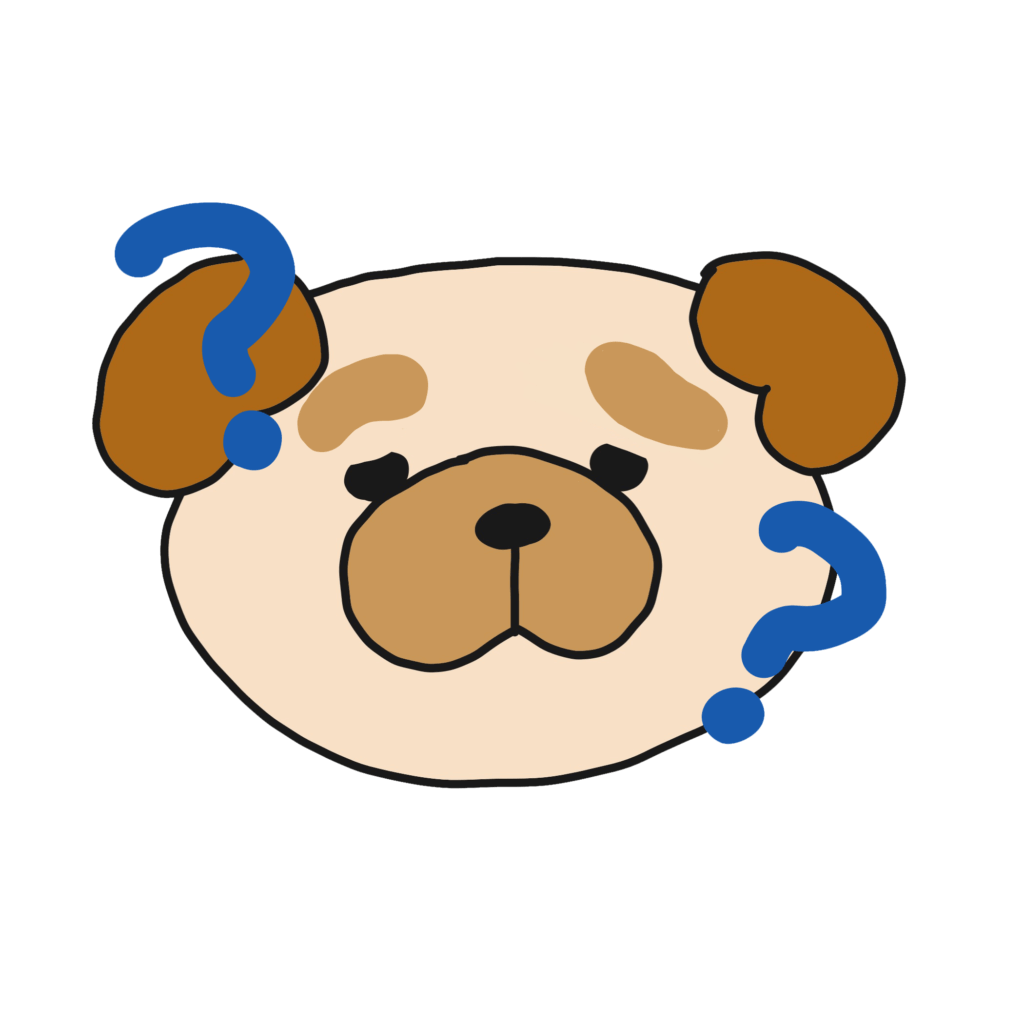
画像にカーソルを乗せて拡大させるのは、実務でも非常に良くある実装です。
そして画像を表示させるには、HTMLの『imgタグ』と、CSSのプロパティ『background-image』の2種類の方法があります。
今回は、両方のケースで画像を拡大する方法を解説していきますが、どちらもCSSのみで実装できます。
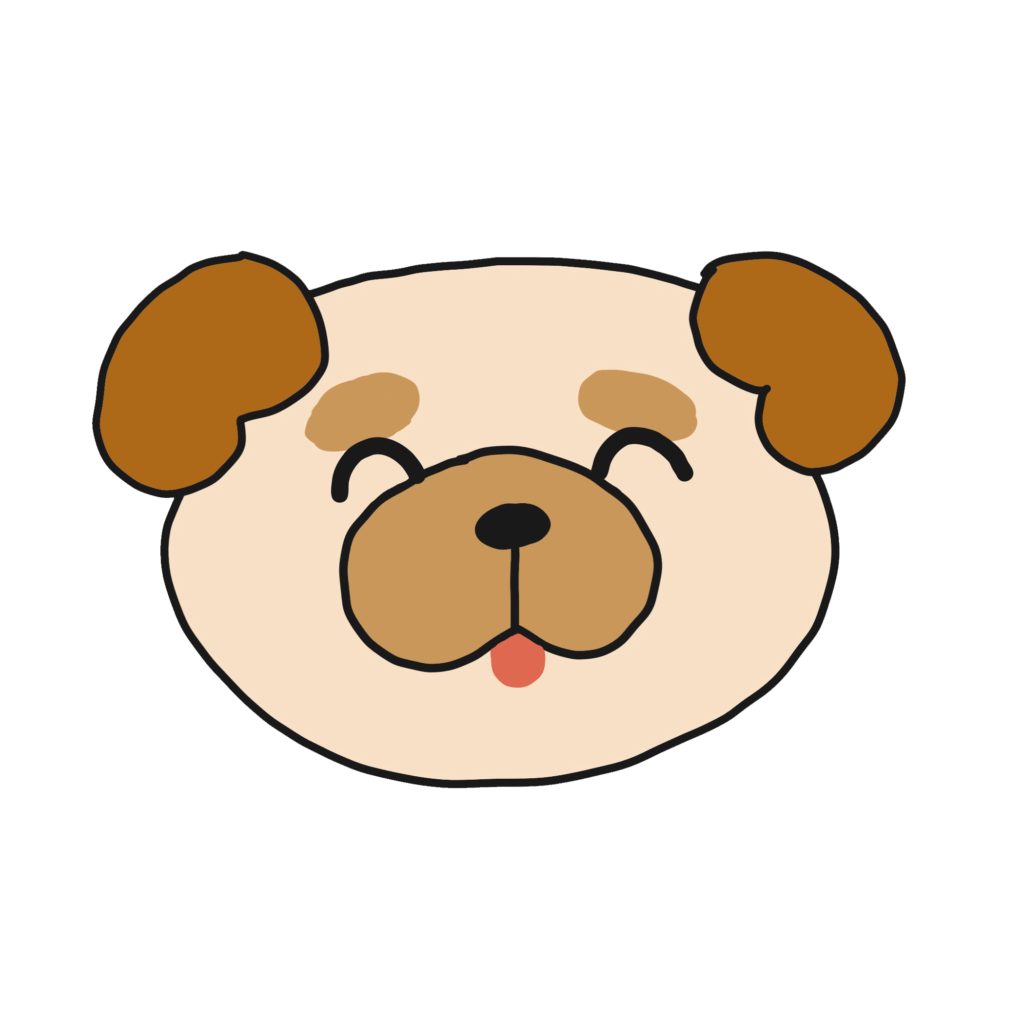
(有料になっていたらすいません🙇♂️)
hover(マウスオーバー)で画像を拡大ズームする方法(imgタグ)
まずは、『imgタグ』を使った場合です。
HTMLのコード
HTMLの書き方はこちら。
<div class="sample-img">
<img src="画像パス" alt="">
</div>
HTMLは、特に変わったところはありません。
CSSのコード
CSSの書き方はこちら。
.sample-img {
cursor: pointer;
max-width: 500px;
overflow: hidden;
width: 100%;
}
.sample-img img {
height: auto;
transition: transform .6s ease; /* ゆっくり変化させる */
}
.sample-img:hover img {
transform: scale(1.1); /* 拡大 */
}
transform: scale(1.1);
で、画像が拡大しています。
これは数字が大きいほど拡大し、1.0未満だと縮小します。
ポイントはoverflow
で、これがないと画像が親要素からはみ出てしまいます。
サンプル(デモ)
こちらがサンプルになります。
See the Pen
hoverで画像拡大 by junpei (@junpei-sugiyama)
on CodePen.
overflowが無いと画像がはみ出る
ちなみに、overflow
が無いとこうなります。
See the Pen
hoverで画像拡大(overflowなし) by junpei (@junpei-sugiyama)
on CodePen.
拡大はしますが・・・枠ごとはみ出てしまいます。
画像の上に文字がある場合
画像の上に文字がある場合は、このようになります。
<div class="sample-img">
<img src="画像パス" alt="">
<p>テキスト</p>
</div>
.sample-img {
cursor: pointer;
max-width: 500px;
overflow: hidden;
position: relative;
width: 100%;
}
.sample-img img {
height: auto;
transition: transform .6s ease; /* ゆっくり変化させる */
}
.sample-img:hover img {
transform: scale(1.1); /* 拡大 */
}
.sample-img p {
align-items: center; /* テキストの中央揃え */
bottom: 0;
color: #fff; /* テキストの色 */
display: flex; /* テキストの中央揃え */
justify-content: center; /* テキストの中央揃え */
left: 0;
margin: auto;
position: absolute;
right: 0;
top: 0;
width: 80%; /* テキストを横幅いっぱいにならないようにする */
}
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
画像だけに黒いマスクをかける
さらに、画像だけ黒いマスクをかけたい場合は、こちらになります。
<div class="sample-img">
<img src="画像パス" alt="">
<p>テキスト</p>
</div>
.sample-img {
cursor: pointer;
max-width: 500px;
overflow: hidden;
position: relative;
width: 100%;
}
.sample-img img {
height: auto;
transition: transform .6s ease; /* ゆっくり変化させる */
}
.sample-img:hover img {
transform: scale(1.1); /* 拡大 */
}
.sample-img p {
align-items: center; /* テキストの中央揃え */
bottom: 0;
color: #fff; /* テキストの色 */
display: flex; /* テキストの中央揃え */
justify-content: center; /* テキストの中央揃え */
left: 0;
margin: auto;
position: absolute;
right: 0;
top: 0;
width: 80%; /* テキストを横幅いっぱいにならないようにする */
z-index: 2;
}
/* マスク */
.sample-img::before {
background: rgba(0, 0, 0, .5); /* マスクの色(黒の50%) */
bottom: 0;
content: '';
height: auto;
left: 0;
opacity: 0; /* 最初は透明(非表示) */
position: absolute;
right: 0;
top: 0;
transition: opacity .6s ease; /* ゆっくりopacityのみへ変化させる */
width: 100%;
z-index: 1;
}
.sample-img:hover::before {
opacity: 1;
}
See the Pen
hoverで画像拡大(テキスト、マスクあり) by junpei (@junpei-sugiyama)
on CodePen.
これは、画像をhoverしたら透過させた黒いマスクをかけて文字を表示させる方法の応用になりますが、テキストとマスクにz-index
を書かないとマスクが反映されなかったり、数値を間違えるとテキストの上にマスクがきてテキストも暗くなるのでご注意下さい。
hover(マウスオーバー)で画像を拡大ズームする方法(background-image)
次は、『background-image』を使った場合です。
HTMLのコード
HTMLの書き方はこちら。
<div class="box">
<div class="box-bg"></div>
</div>
今度は『imgタグ』ではなく、背景画像の『background-image』で画像を表示しています。
背景画像は.box-bg
に表示させます。
CSSのコード
CSSの書き方はこちら。
.box {
cursor: pointer;
max-width: 500px;
overflow: hidden;
position: relative;
width: 100%;
}
.box-bg {
background-image: url(画像パス);
background-position: center;
background-repeat: no-repeat;
background-size: cover;
height: 350px;
transition: transform .6s ease;
width: 100%;
}
.box:hover .box-bg {
transform: scale(1.1);
}
サンプル(デモ)
See the Pen
hoverでbackground-imageを拡大 by junpei (@junpei-sugiyama)
on CodePen.
画像の上に文字がある場合
画像の上に文字がある場合は、このようになります。
<div class="box">
<p>テキスト</p>
<div class="box-bg"></div>
</div>
.box {
cursor: pointer;
max-width: 500px;
overflow: hidden;
position: relative;
width: 100%;
}
.box-bg {
background-image: url(画像パス);
background-position: center;
background-repeat: no-repeat;
background-size: cover;
height: 350px;
transition: transform .6s ease;
width: 100%;
}
.box:hover .box-bg {
transform: scale(1.1);
}
.box p {
align-items: center; /* テキストの中央揃え */
bottom: 0;
color: #fff; /* テキストの色 */
display: flex; /* テキストの中央揃え */
justify-content: center; /* テキストの中央揃え */
left: 0;
margin: auto;
pointer-events: none;
position: absolute;
right: 0;
top: 0;
width: 80%; /* テキストを横幅いっぱいにならないようにする */
z-index: 1;
}
See the Pen
hoverでbackground-imageを拡大(テキストあり) by junpei (@junpei-sugiyama)
on CodePen.
画像だけに黒いマスクをかける
さらに、画像だけ黒いマスクをかけたい場合は、こちらになります。
<div class="box">
<p>テキスト</p>
<div class="box-bg"></div>
</div>
.box {
cursor: pointer;
max-width: 500px;
overflow: hidden;
position: relative;
width: 100%;
}
.box-bg {
background-image: url(画像パス);
background-position: center;
background-repeat: no-repeat;
background-size: cover;
height: 350px;
transition: transform .6s ease;
width: 100%;
}
.box:hover .box-bg {
transform: scale(1.1);
}
.box p {
align-items: center; /* テキストの中央揃え */
bottom: 0;
color: #fff; /* テキストの色 */
display: flex; /* テキストの中央揃え */
justify-content: center; /* テキストの中央揃え */
left: 0;
margin: auto;
position: absolute;
right: 0;
top: 0;
width: 80%; /* テキストを横幅いっぱいにならないようにする */
z-index: 2;
}
/* マスク */
.box::before {
background: rgba(0, 0, 0, .5); /* マスクの色(黒の50%) */
bottom: 0;
content: '';
height: auto;
left: 0;
opacity: 0; /* 最初は透明(非表示) */
position: absolute;
right: 0;
top: 0;
transition: opacity .6s ease; /* ゆっくりopacityのみへ変化させる */
width: 100%;
z-index: 1;
}
.box:hover::before {
opacity: 1;
}
See the Pen
hoverでbackground-imageを拡大(テキスト&マスクあり) by junpei (@junpei-sugiyama)
on CodePen.
ポイントは以下になります。
- overflowで親要素からはみ出させないようにする
- z-indexでマスクとテキストの順番を指定する
画像の拡大はスマホでは効果が薄い
今回の方法はスマホでも効果はありますが、この機能はパソコンでのWebサイト閲覧に特化していて、スマホやタブレットでの使用には適していません。
タッチ操作が主流のスマホやタブレットではマウスオーバーの概念がないため、この機能は動作しません。リンク先が設定されている場合、タップすると画像が拡大表示されたあと、即座にリンク先のページに遷移してしまいます。
パソコンユーザー、特に年配層の方々にとっては、魅力的な演出として受け入れられやすいでしょう。しかし、現代のWebサイトはスマホでのアクセスが大半を占めるため、この機能がユーザーに体験される機会は限定的かもしれません。
Web制作で導入を検討する際は、
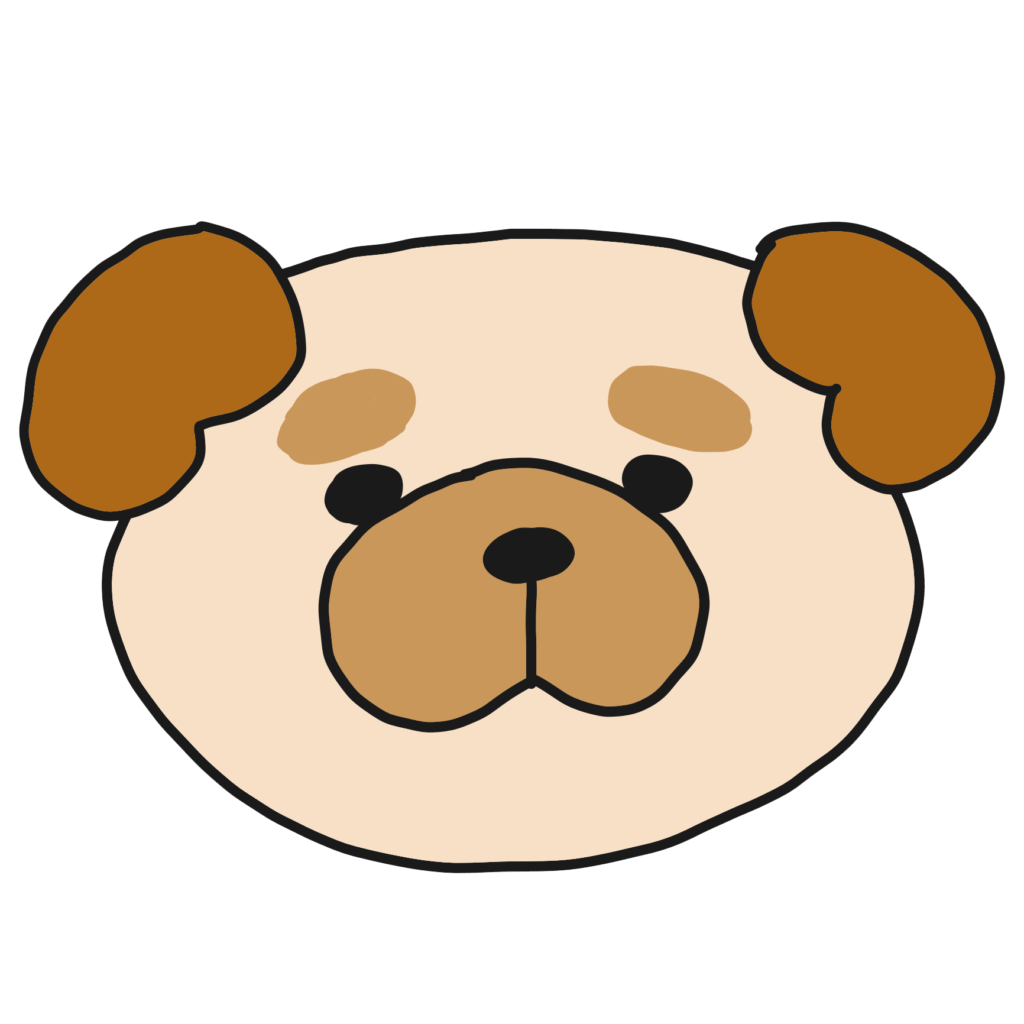
と、クライアントに伝えた上で実装すると、安心かも知れません。
まとめ:hoverで画像を拡大ズームさせる方法は2種類ある!
今回は、Webサイトに動きを与える要素として、hover(マウスオーバー)で画像を拡大する方法を解説しました。
ブログ記事一覧やコンテンツ一覧ページなど、リンクが並ぶレイアウトでよく活用されるこの手法は、ユーザーにとって視覚的な分かりやすさを向上させ、クリックを促す効果が期待できます。
実装も比較的容易でCSSの設定だけで実現できるため、コーディング初心者でも気軽に挑戦できます。ただし、拡大率などの設定を過剰にすると、かえって使いにくくなる可能性もあるため注意が必要です。
リンクに動きを加えたい、Webサイトにアクセントをつけたいと考えている方は、ぜひこの効果を試してみてはいかがでしょうか。
実務でもよくある実装だと思うので、ブクマしていつでもコピペ出来るようにしておくと便利かと思います。
以上になります。
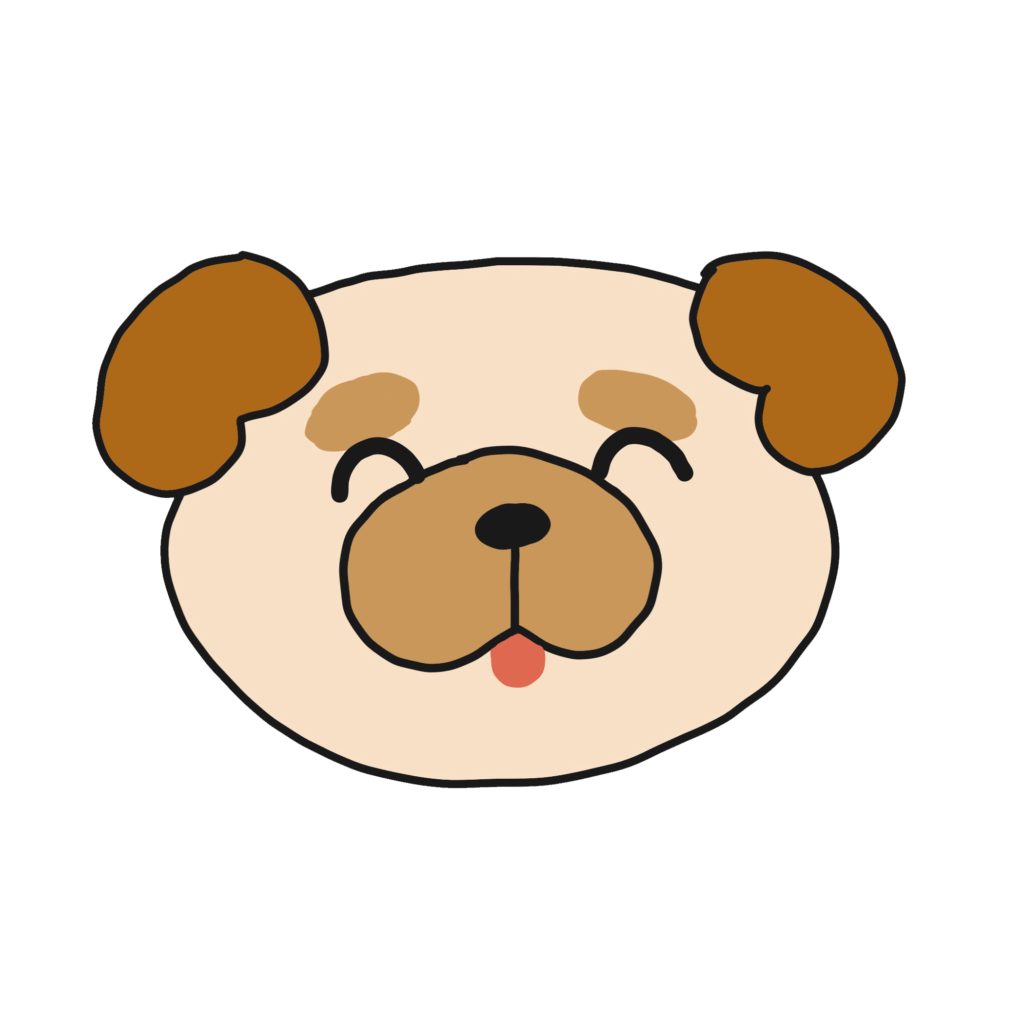