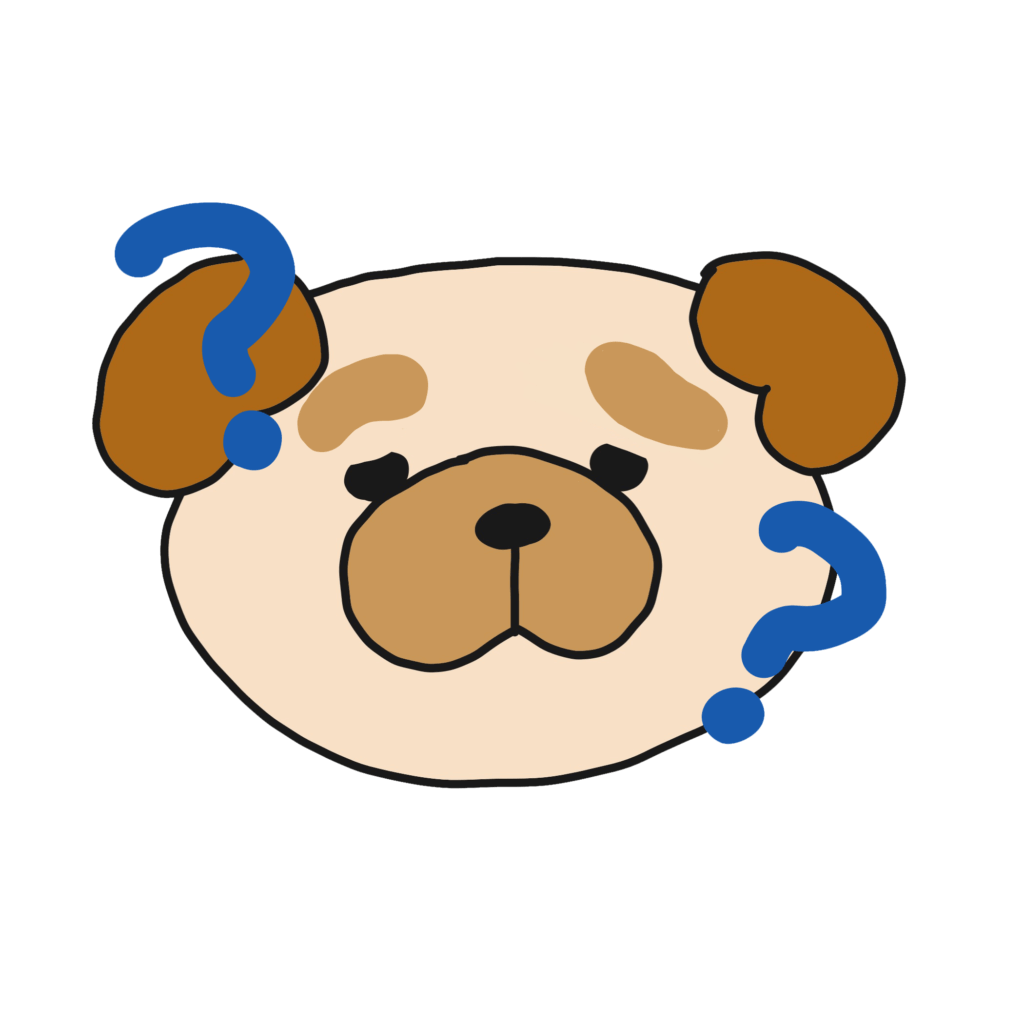
GSAPのアニメーションは特に何もしないとページを開いた瞬間に始まります。
そしてスクロールをして画面内に入ったらアニメーションを開始させることもできます。
その方法は以下の記事で解説しています。
-
【GSAP】ScrollTriggerの使い方とスクロールアニメーションのサンプルを紹介
続きを見る
そして今回はボタンなどのクリックやマウスカーソルのホバーでアニメーションを開始させる方法を解説します。
GSAPを使ったことがないという人は以下の記事を参照下さい。
-
GSAPとは?基本的な使い方とアニメーションのサンプル付きで解説
続きを見る
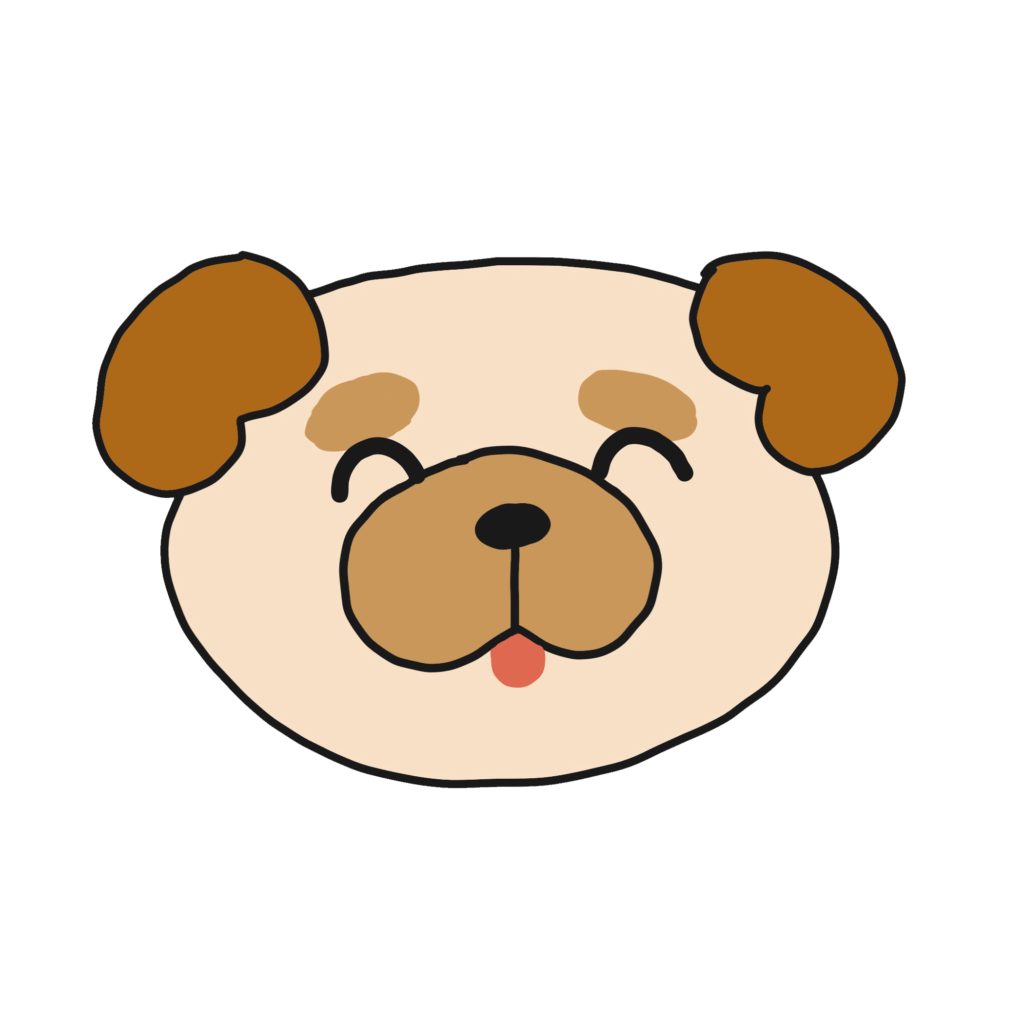
(有料になっていたらすいません🙇♂️)
GSAPでクリックしたらアニメーションを開始させる方法
まずはボタンをクリックしたら、ボックスの要素がアニメーションを開始する方法を解説します。
ボタンがclassの場合とidの場合で書き方が少し違うので、両方解説します。
CSSはただの調整用なので割愛します。
classの場合
ボタンにclassが付いている場合のHTMLはこちらです。
<button class="js-button">ボタン</button>
<div class="box"></div>
JavaScriptはこちらです。
// ボタンのクリックイベントを取得
document.querySelector(".js-button").addEventListener("click", function () {
// .box の背景色を赤に変更し、GSAPアニメーションを設定
gsap.to(".box", {
backgroundColor: "tomato", // 背景色を赤 (tomato) に変更
rotate: 720, // 要素を720度回転
scale: 2, // 要素を2倍に拡大
x: 200, // x軸方向に200px移動
y: 20, // y軸方向に20px移動
transformOrigin: "left bottom", // 変形の基点を要素の左下に設定
borderRadius: "50%", // 角丸にする(円形にする)
duration: 2, // アニメーションの時間(2秒)
});
});
色々書いていますが、重要なのは最初とアニメーションさせたい要素を指定する".box"
だけです。
まとめるとこうなります。
// ボタンのクリックイベントを取得
document.querySelector("クリックする要素").addEventListener("click", function () {
// GSAPアニメーションを設定
gsap.to("アニメーションさせる要素", {
// アニメーション内容
});
});
サンプルはこちらです。
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
右下のRerunをクリックすればリセットされます。
idの場合
ボタンにidが付いている場合のHTMLはこちらです。
<button id="js-button">ボタン</button>
<div class="box"></div>
JavaScriptはこちらです。
// ボタンのクリックイベントを取得
document.getElementById("js-button").addEventListener("click", function () {
// .box の背景色を赤に変更し、GSAPアニメーションを設定
gsap.to(".box", {
backgroundColor: "tomato", // 背景色を赤 (tomato) に変更
rotate: 720, // 要素を720度回転
scale: 2, // 要素を2倍に拡大
x: 200, // x軸方向に200px移動
y: 20, // y軸方向に20px移動
transformOrigin: "left bottom", // 変形の基点を要素の左下に設定
borderRadius: "50%", // 角丸にする(円形にする)
duration: 2, // アニメーションの時間(2秒)
});
});
classの時との違いは最初の行だけですが、getElementById("#js-button")
ではなくgetElementById("js-button")
なので注意しましょう。
サンプルはこちらです。
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
classでもう一度クリックしたら逆再生してアニメーションを戻す方法
classでボタンをもう一度クリックしたらアニメーションを逆再生するように戻すには、JavaScriptを以下のように書きます。
let isAnimated = false;
let tl;
document.querySelector(".js-button").addEventListener("click", function () {
if (!isAnimated) {
tl = gsap.timeline(); // Timelineインスタンスを作成
tl.to(".box", {
backgroundColor: "tomato",
rotate: 720,
scale: 2,
x: 200,
y: 20,
transformOrigin: "left bottom",
borderRadius: "50%",
duration: 2,
});
isAnimated = true;
} else {
tl.reverse(); // アニメーションを逆再生
isAnimated = false;
}
});
サンプルはこちらです。
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
idでもう一度クリックしたら逆再生してアニメーションを戻す方法
idでボタンをもう一度クリックしたらアニメーションを逆再生するように戻すには、JavaScriptを以下のように書きます。
let tl = gsap.timeline({ paused: true });
document.getElementById("js-button").addEventListener("click", function () {
if (tl.progress() < 1) {
tl.play();
} else {
tl.to(".box", {
backgroundColor: "",
rotate: 0,
scale: 1,
x: 0,
y: 0,
transformOrigin: "left top",
borderRadius: "0",
duration: 2,
}).reverse();
}
});
tl.to(".box", {
backgroundColor: "tomato",
rotate: 720,
scale: 2,
x: 200,
y: 20,
transformOrigin: "left bottom",
borderRadius: "50%",
duration: 2,
});
サンプルはこちらです。
See the Pen
GSAP(クリック・id・) by junpei (@junpei-sugiyama)
on CodePen.
GSAPでマウスカーソルをホバーしたらアニメーションを開始させる方法
今度はボタンにマウスカーソルをホバーしたら、ボックスの要素がアニメーションを開始する方法を解説します。
これもclassとidの違いは先ほどと同じで、CSSはただの調整用なので割愛します。
classの場合
ボタンにclassが付いている場合のHTMLはこちらです。
<button class="js-button">ボタン</button>
<div class="box"></div>
JavaScriptはこちらです。
// ボタンのホバーイベントを取得
document.querySelector(".js-button").addEventListener("mouseover", function () {
// .box の背景色を赤に変更し、GSAPアニメーションを設定
gsap.to(".box", {
backgroundColor: "tomato", // 背景色を赤 (tomato) に変更
rotate: 720, // 要素を720度回転
scale: 2, // 要素を2倍に拡大
x: 200, // x軸方向に200px移動
y: 20, // y軸方向に20px移動
transformOrigin: "left bottom", // 変形の基点を要素の左下に設定
borderRadius: "50%", // 角丸にする(円形にする)
duration: 2, // アニメーションの時間(2秒)
});
});
クリックイベントとの違いは、最初の"click"
が"mouseover"
になっただけです。
サンプルはこちらです。
See the Pen
GSAP(ホバー・class) by junpei (@junpei-sugiyama)
on CodePen.
idの場合
ボタンにidが付いている場合のHTMLはこちらです。
<button id="js-button">ボタン</button>
<div class="box"></div>
JavaScriptはこちらです。
// ボタンのホバーイベントを取得
document.getElementById("js-button").addEventListener("mouseover", function () {
// .box の背景色を赤に変更し、GSAPアニメーションを設定
gsap.to(".box", {
backgroundColor: "tomato", // 背景色を赤 (tomato) に変更
rotate: 720, // 要素を720度回転
scale: 2, // 要素を2倍に拡大
x: 200, // x軸方向に200px移動
y: 20, // y軸方向に20px移動
transformOrigin: "left bottom", // 変形の基点を要素の左下に設定
borderRadius: "50%", // 角丸にする(円形にする)
duration: 2, // アニメーションの時間(2秒)
});
});
これもクリックイベントとの違いは、最初の"click"
が"mouseover"
になっただけです。
サンプルはこちらです。
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
classでホバーを外したら逆再生してアニメーションを戻す方法
classでボタンからホバーを外したらアニメーションを逆再生するように戻すには、JavaScriptを以下のように書きます。
let tl = gsap.timeline({ paused: true });
// ボタンの要素を取得
let button = document.querySelector(".js-button");
// アニメーションの設定
tl.to(".box", {
backgroundColor: "tomato",
rotate: 720,
scale: 2,
x: 200,
y: 20,
transformOrigin: "left bottom",
borderRadius: "50%",
duration: 2,
});
// ボタンのホバーイベントを設定
button.addEventListener("mouseover", function () {
tl.play();
});
// ボタンのマウスアウトイベントを設定
button.addEventListener("mouseout", function () {
tl.reverse();
});
サンプルはこちらです。
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
idでホバーを外したら逆再生してアニメーションを戻す方法
idでボタンからホバーを外したらアニメーションを逆再生するように戻すには、JavaScriptを以下のように書きます。
let tl = gsap.timeline({ paused: true });
// ボタンの要素を取得
let button = document.getElementById("js-button");
// アニメーションの設定
tl.to(".box", {
backgroundColor: "tomato",
rotate: 720,
scale: 2,
x: 200,
y: 20,
transformOrigin: "left bottom",
borderRadius: "50%",
duration: 2,
});
// ボタンのホバーイベントを設定
button.addEventListener("mouseover", function () {
tl.play();
});
// ボタンのマウスアウトイベントを設定
button.addEventListener("mouseout", function () {
tl.reverse();
});
サンプルはこちらです。
See the Pen
Untitled by junpei (@junpei-sugiyama)
on CodePen.
まとめ
今回はGSAPのアニメーションをボタンのクリックやマウスカーソルのホバーで開始させる方法を解説しました。
これで当ブログでは、
- ページを開いた瞬間にアニメーション開始(デフォルト)
- スクロールして画面内に入ったらアニメーション開始
- ボタンなどクリックでアニメーション開始
- ボタンなどホバーでアニメーション開始
と、4種類のアニメーション開始のタイミングを解説しました。
これらを使いこなせるようになると出来ることの幅が広がると思います。
以上になります。
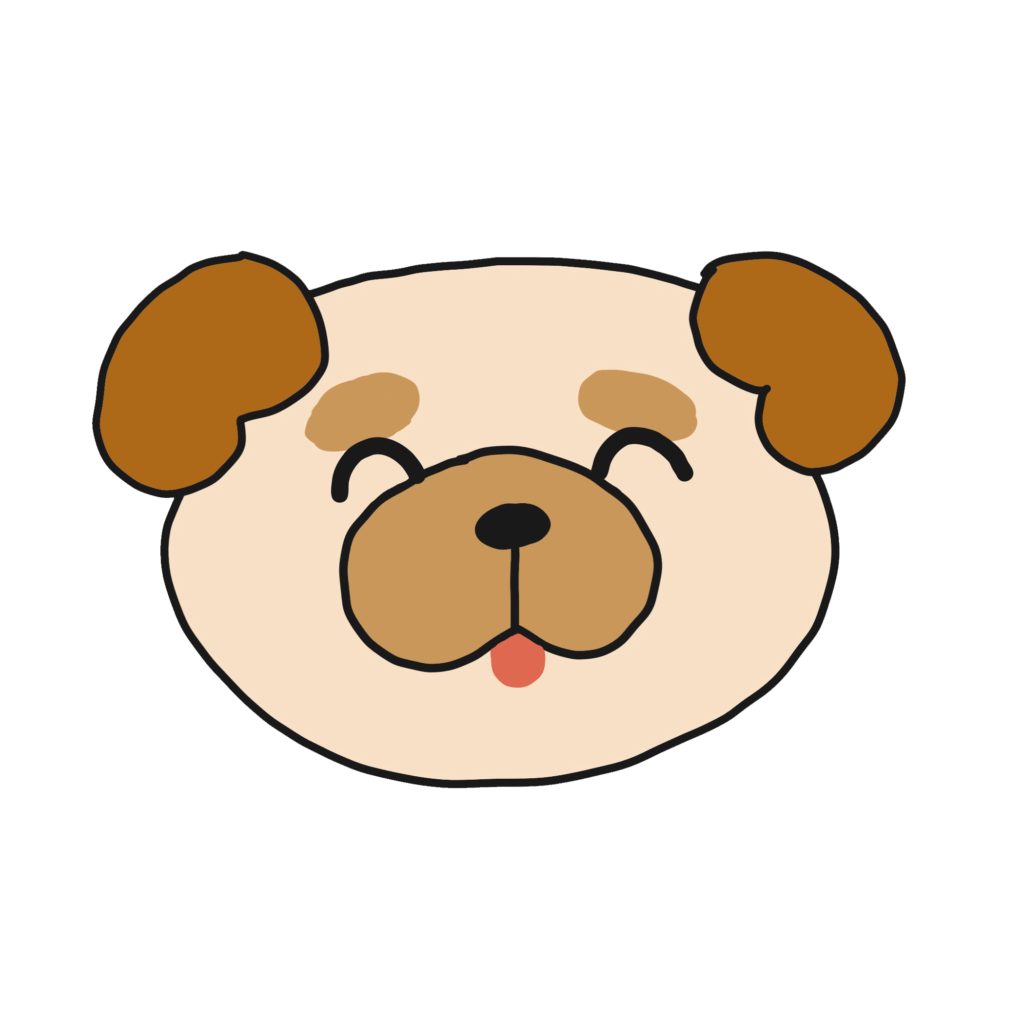